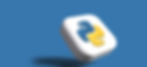
Introduction:
String manipulation is a fundamental aspect of programming, and Python provides a powerful and flexible tool for handling string formatting: the format() method. With its extensive capabilities, the format() method enables you to create dynamic, readable, and expressive strings. In this article, we will explore the advanced features of the format() method and provide illustrative examples to showcase its versatility.
The Basics of format(): The format() method allows you to insert values into a string by using placeholders represented by curly braces {}. You can then specify the values to be inserted either directly or by referencing variables. Here's a simple example:
name = "Alice"
age = 25
message = "My name is {} and I am {} years old.".format(name, age)
print(message)
Output:
My name is Alice and I am 25 years old.
Positional and Keyword Arguments: The format() method supports two methods of providing arguments: positional and keyword arguments. Positional arguments are referenced by their order, while keyword arguments are referenced by their names. Let's see an example of both:
name = "Bob"
age = 30
message = "My name is {0} and I am {1} years old.".format(name, age)
print(message)
message = "My name is {name} and I am {age} years old.".format(name="Bob", age=30)
print(message)
Output:
My name is Bob and I am 30 years old.
My name is Bob and I am 30 years old.
Formatting Types: The format() method provides various formatting options to control how the values are displayed. Some commonly used formatting types include:
Number Formatting:
pi = 3.141592653589793
message = "The value of pi is approximately {:.2f}".format(pi)
print(message)
Output:
The value of pi is approximately 3.14
Accessing Elements of a Dictionary or List: The format() method also allows you to access individual elements of a dictionary or list using indexing or key names. Here's an example:
person = {"name": "Sarah", "age": 28}
message = "My name is {0[name]} and I am {0[age]} years old.".format(person)
print(message)
fruits = ["apple", "banana", "cherry"]
message = "I like {1[1]} and {1[2]}.".format("fruits", fruits)
print(message)
Output:
My name is Sarah and I am 28 years old.
I like banana and cherry.
Conclusion: The format() method in Python offers a rich set of features for advanced string formatting. By understanding the basics, positional and keyword arguments, formatting types, and accessing elements of dictionaries or lists, you can manipulate strings with ease and precision. This versatility makes the format() method an indispensable tool for any Python developer.
With the knowledge gained from this article, you're well-equipped to harness the full potential of the format() method and take your string formatting skills to the next level in Python. Happy coding!